객체
배열
- ‘인덱스’와 ‘요소’
- 접근 - 배열이름[인덱스]
- <script> var array =[‘사과’, ‘바나나’, ‘망고’, ‘딸기’]; alert( array[0] ) alert( array[1] ) </script>
객체
- 중괄호({})를 사용하여 생성
- 키와 요소
- 접근 - 객체이름[’키’] 또는 이름.키
- product[’유형’]
- product.유형
<script> var product = { 제품명 : ‘7D 건조 망고‘, 유형 : ‘당절임‘, 성분: ‘망고, 설탕, 메타중아황산나트륨’, 원산지: ‘필리핀‘ }; </script>
속성과 메서드
- 객체의 속성이 가질 수 있는 자료형
- 배열의 내부에 저장된 각각의 정보를 ‘요소’라고 부름
- 객체 내부에 저장된 각각의 정보를 ‘속성(property)’이라고 함
- 사물의 특성
- 객체의 속성 중 함수 자료형인 속성은 특별히 메서드(method)라 부름
- 사물의 동작
<script> var object = { number : 273, string : ‘ha ha’, boolean: ture, array : [52, 273, 103, 32], method : function ( ) { } }; </script>
<script> var person = { name : ‘jaeyeong’, eat : function( food ) { alert(‘eat function’); } }; person.eat( ); </script>
- this 는 자기 자신, 파이썬에서 self와 같음
- 메서드 내에서 자기 자신(객체)이 가진 속성을 출력(속성에 접근)하고 싶을 때 자신이 가진 속성임을 분명하기 표시
- 자바스크립트에서는 this 키워드를 생략할 수 없음
<script>
var person = {
name : ‘jaeyeong’,
eat : function( food ) {
alert( this.name + ‘이가 ’ + food +’를(을) 먹습니다.’ );
}
};
person.eat(‘밥’);
</script>
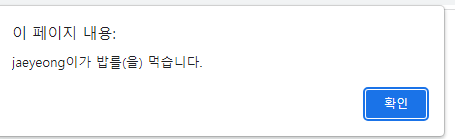
속성 존재 여부 확인
- 객체에 없는 속성에 접근하면 undefined 자료형이 나옴.
- 따라서 조건문으로 접근하려는 속성이 undefined인지 아닌지 확인하면 속성 존재 여부를 확인할 수 있음
<script>
const obj = {
name:'혼자 공부하는 파이썬',
price:18000,
publisher:'한빛미디어'
}
if(obj.name != undefined) {
console.log('name 속성이 있음')
} else {
console.log('name 속성이 없음')
}
</script>

객체와 반복문
- 배열은 단순 for 문과 for in 문으로 요소에 쉽게 접근 가능.
- 객체는 단순 for 문으로 객체의 속성을 살펴 보는 것은 불가능하여
- for in 문을 사용 함
<script>
var product = {
name : ‘MicroSoft Visual Studio 2015 Ultimate’,
price : ‘1,500,000원’,
language : ‘한국어’,
supportOS : ‘Win32/64’,
subscription : true
};
var output = ‘’;
for( var key in product ) {
output += key + ‘:’ + product[key] + ‘\\n’;
}
alert(output)
</script>
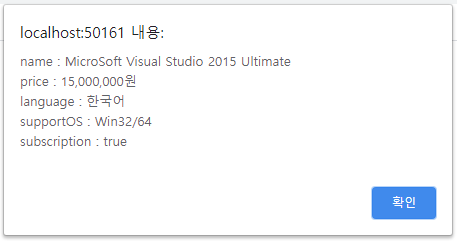
객체 관련 키워드
- 객체 관련 in 키워드와 with 키워드에 대해 살펴 봄
in 키워드
- 객체 내에 해당 속성이 있는지 확인
<script>
var student = {
이름 : ‘이재영’,
국어: 92, 수학 : 98, 영어:96, 과학:98
};
var output =‘’;
output += “ ’이름’ in student : “ + ( ‘이름’ in student ) + ‘\\n’;
output += “ ’성별’ in student : “ + ( ‘성별’ in student );
alert(output);
</script>
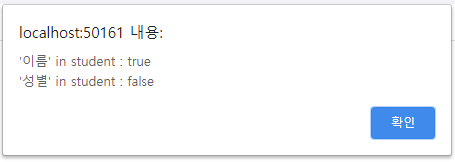
with 키워드
<script>
var student = {
이름 : ‘이재영’,
국어점수: 92, 수학점수 : 98, 영어점수:96, 과학점수:98
};
var output =‘’
with( student ) {
output += ‘이름: ‘ + 이름 + ‘\\n’;
output += ‘국어: ‘ + 국어점수 + ‘\\n’;
output += ‘수학: ‘ + 수학점수 + ‘\\n’;
output += ‘영어: ‘ + 영어점수 + ‘\\n’;
output += ‘과학: ‘ + 과학점수+ ‘\\n’;
output += ‘총점: ‘ + (국어점수 + 수학점수 + 영어점수 + 과학점수);
}
alert(output);
</script>
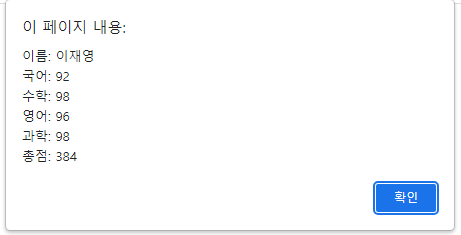
- 객체의 속성을 출력할 때 식별자(student)를 여러 번 사용하니 번거로움
- with( 객체 ) { <코드> }
- 객체를 지정하면 지정된 블럭 내에서 일일이 객체 명을 지정하지 않고 속성이름만 가지고 접근 가능
객체의 속성 추가와 제거
객체에 속성 추가
빈 객체 선언
<script>
var student = { };
</script>
동적으로 속성 추가
<script>
var student = { };
student.이름 = ‘김성필‘;
student.취미 = ‘낚시‘;
student.특기 = ‘드라이브‘;
student.장래희망 = ‘사장’;
</script>
동적으로 메서드 추가
<script>
var student = { };
student.이름 = ‘김성필‘;
student.취미 = ‘낚시‘;
student.특기 = ‘드라이브‘;
student.장래희망 = ‘사장’;
student.toString = function( ) {
var output = ‘’;
for( var key in this ) {
if (key != ‘toString’) {
output += key + ‘\\t’ + this[key] + ‘\\n’;
}
}
return output;
}
alert( student.toString() );
</script>
객체에 속성 제거
<script>
var student = { };
student.이름 = ‘김성필‘;
student.취미 = ‘낚시‘;
student.특기 = ‘드라이브‘;
student.장래희망 = ‘사장’;
student.toString = function( ) {
var output = ‘’;
for( var key in this ) {
if (key != ‘toString’) {
output += key + ‘\\t’ + this[key] + ‘\\n’;
}
}
return output;
}
alert( student.toString( ) );
delete( student.특기);
alert( student.toString( ) );
</script>
메서드 간단 선언 구문
<script>
const pet = {
name : '구름',
eat : function(food) {
console.log(this.name +'은/는 ' + food +'을/를 먹습니다.')
}
}
pet.eat('밥')
</script>
<script>
const pet = {
name : '구름',
eat(food) {
console.log(this.name +'은/는 ' + food +'을/를 먹습니다.')
}
}
pet.eat('밥')
</script>
Number 객체
- 소수점 이하 몇 자리까지 남길 것인지 결정
<script>
let n = 123.456789
n.toFixed(2)
console.log(n) //⇒ n은 바뀌지 않음
n = n.toFixed(2) //=> 이렇게 해야 바뀜
console.log(n)
</script>
NaN과 Infinity 확인하기 : isNaN( ), isFinity( )
Number.isNaN( ), Number.isFinity( )
<script>
const n = Number('숫자로 변환 불가')
console.log(n)
console.log(Number.isNaN(n))
</script>

<script>
const n = 10 / 0
const m = -10 / 0
console.log(n)
console.log(m)
console.log(Number.isFinite(n))
console.log(Number.isFinite(m))
console.log( n === Infinity || n === -Infinity)
</script>
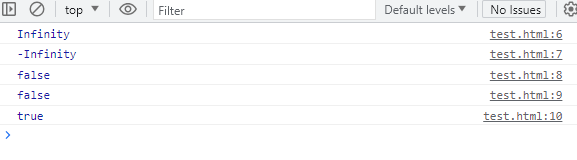
String 객체
문자열 양쪽 끝의 공백 없애기 : trim( )
<script>
const stringA = '\\n 메시지를 입력하다 보니 '
console.log('kim')
console.log('lee')
console.log(stringA)
console.log('trim() 적용')
console.log(stringA.trim())
</script>
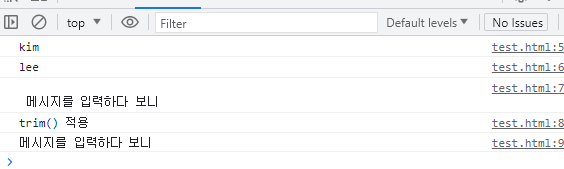
문자열을 특정 기호로 자르기: split( )
<script>
const string = '수박, 사과, 복숭아, 참외'
console.log(string.split(','))
</script>
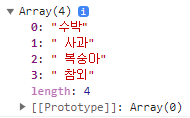
<script>
const string = '수박, 사과, 복숭아, 참외'
console.log(string.split(',').map(function(value){
return value.trim()
}))
</script>
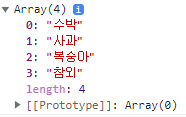
<script>
const string = '수박, 사과, 복숭아, 참외'
console.log(string.split(',').map((value)=>value.trim()))
</script>
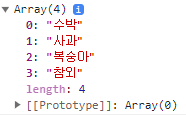
Quiz - 주어진 문자열의 각 행을 배열로 바꾸는 코드를 작성
let input = `
일자, 달러, 엔, 유로
02, 1141.8, 1097.46, 1262.37
03, 1148.7, 1111.36, 1274.65
04, 1140.6, 1107.81, 1266.58
`
// 1
<script>
let input = `
일자, 달러, 엔, 유로
02, 1141.8, 1097.46, 1262.37
03, 1148.7, 1111.36, 1274.65
04, 1140.6, 1107.81, 1266.58
`
let arr = input.split('\\n').map((value)=>value.trim())
console.log(arr);
let filtered_lines = arr.filter(function(value){
return Boolean(value)
})
console.log(filtered_lines)
let output = filtered_lines.map(function(value){
return value.split(',')
})
console.log(output)
</script>
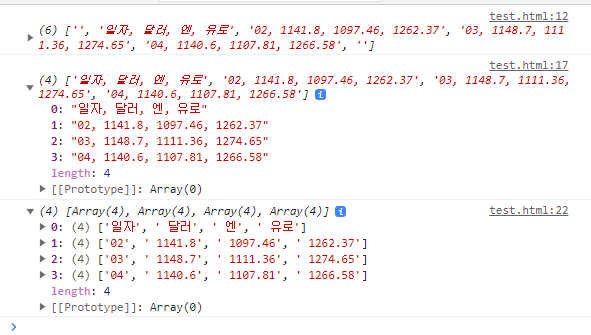
// 2
<script>
let input = `
일자, 달러, 엔, 유로
02, 1141.8, 1097.46, 1262.37
03, 1148.7, 1111.36, 1274.65
04, 1140.6, 1107.81, 1266.58
`
let lines = input.trim()
console.log(lines)
lines = lines.split('\\n')
console.log(lines)
lines = lines.map(function(value){
return value.trim()
})
console.log(lines)
lines = lines.map(function(value){
return value.split(',')
})
console.log('최종 결과물 : ', lines)
// console.log('최종 결과물 : ', input.trim().split('\\n').map((value) => value.trim()).map((value)=>value.split(',')))
</script>
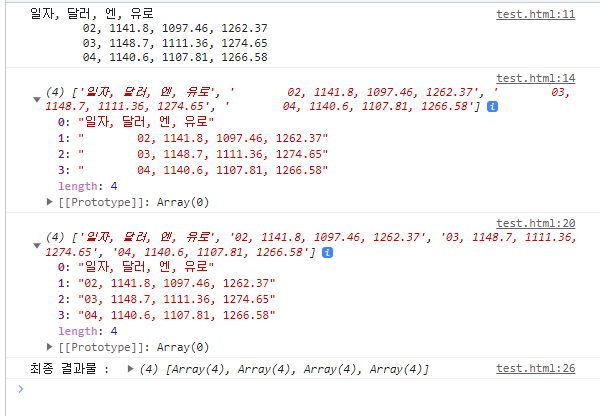
JSON 객체
- 인터넷에서 문자열로 데이터를 주고 받을 때는 CSV, XML, CSON 등의 다양한 자료 표현 방식이 있음
- 현재 가장 많이 사용되는 자료 표현 방식은 JSON 임
- JSON은 JavaScript Object Notation의 약자로 자바스크립트의 객체처럼 자료를 표현하는 방식
- JSON를 사용하여 ‘책’ 객체를 표현
- 하나의 자료를 표현 하는 예
{
"name":"혼자 공부하는 자바스크립트",
"price":18000,
"publisher":"한빛미디어"
}
[{
"name":"혼자 공부하는 자바스크립트",
"price":18000,
"publisher":"한빛미디어"
},
{
"name":"HTML5 웹 프로그래밍 입문",
"price":26000,
"publisher":"한빛미디어"
}]
- JSON은 “자바스크립트의 배열과 객체 표기 방식으로 표현된 데이터”임 - 추가적인 제약 조건이 있음
- 값을 표현할 때는 문자열, 숫자, 불 자료형만 사용(함수 등은 사용 불가)
- 문자열은 반드시 큰따옴표로 만듦
- 키(key)에도 반드시 따옴표를 붙여야 함
JSON.stringify( )
- 자바스크립트 객체를 JSON 문자열로 변환
<script>
const data = [{
name :'혼자 공부하는 자바스크립트',
price : 18000,
publisher :'한빛미디어'
},{
name : 'HTML5 웹 프로그래밍 입문',
price : 26000,
publisher :'한빛미디어'
}]
console.log(JSON.stringify(data))
console.log(JSON.stringify(data, null, 2))
</script>
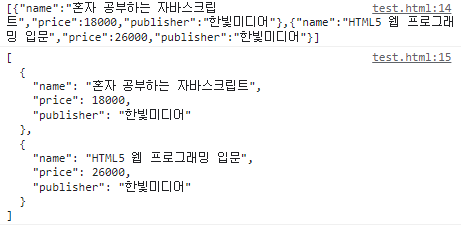
JSON.parse( )
- JSON 문자열을 자바스크립트 자료형으로 변환
<script>
const data = [{
name :'혼자 공부하는 자바스크립트',
price : 18000,
publisher :'한빛미디어'
},{
name : 'HTML5 웹 프로그래밍 입문',
price : 26000,
publisher :'한빛미디어'
}]
const json = JSON.stringify(data)
console.log(json)
console.log(JSON.parse(json))
</script>
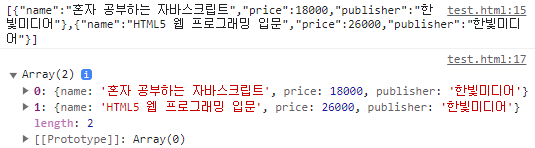
외부 스크립트 파일 읽어 들이기
<html>
<head>
<script src = "test.js"></script>
<script>
console.log('# main.html의 script 태그')
console.log('sample의 값:', sample)
</script>
</head>
<body>
</body>
</html>
console.log('# test.js 파일')
const sample = 10
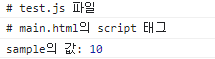
객체와 배열을 사용한 데이터 관리
학생들의 성적 총점과 평균을 계산하는 예제
- 객체의 필요한 속성을 추출하는 작업을 ‘추상화’라 함
<script>
var student0 = { 이름: ‘윤인성’, 국어:87, 수학:98, 영어:88, 과학:95 };
var student1 = { 이름: ’연하진’, 국어:92, 수학:98, 영어:96, 과학:98 };
var student2 = { 이름: ‘구지연’, 국어:76, 수학:80, 영어:44, 과학:66 };
var student3 = { 이름: ‘구숙정’, 국어:78, 수학:80, 영어:92, 과학:69 };
</script>
객체가 별로도 존재하면 관리가 어려움 → 각 객체를 배열의 요소로 할당
<script>
var students = Array( );
students.push( { 이름: ‘윤인성’, 국어:87, 수학:98, 영어:88, 과학:95 } );
students.push( { 이름: ’연하진’, 국어:92, 수학:98, 영어:96, 과학:98 } );
students.push( { 이름: ‘구지연’, 국어:76, 수학:80, 영어:44, 과학:66 } );
students.push( { 이름: ‘구숙정’, 국어:78, 수학:80, 영어:92, 과학:69 } );
</script>
- 배열 students에 4개의 요소가 생성됨
- 각각의 객체(여기서는 배열의 각 요소)에 getSum( )과 getAverage( ) 메서드를 부여
for (var i in students) {
students[i].getSum = function( ) {
return this.국어 + this.수학 + this.영어 + this.과학;
};
students[i].getAverage = function( ) {
return this.getSum( ) / 4;
};
}
<script>
var students = Array();
students.push( { 이름: '윤인성', 국어:87, 수학:98, 영어:88, 과학:95 } );
students.push( { 이름: '연하진', 국어:92, 수학:98, 영어:96, 과학:98 } );
students.push( { 이름: '구지연', 국어:76, 수학:80, 영어:44, 과학:66 } );
students.push( { 이름: '구숙정', 국어:78, 수학:80, 영어:92, 과학:69 } );
for (var i in students) {
students[i].getSum = function( ) {
return this.국어 + this.수학 + this.영어 + this.과학;
};
students[i].getAverage = function( ) {
return this.getSum( ) / 4;
};
}
var output = '이름\\t총점\\t평균\\n';
for ( var i in students) {
with ( students[i] ) {
output+= 이름 + '\\t' + getSum( ) +'\\t' + getAverage( ) + '\\n';
}
}
alert(output);
</script>
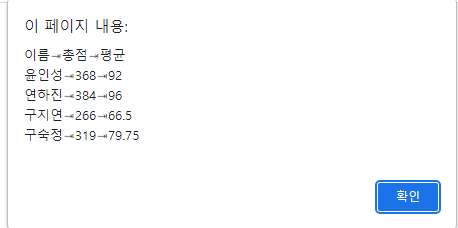
<script>
var students = Array();
students.push( { 이름: '윤인성', 국어:87, 수학:98, 영어:88, 과학:95 } );
students.push( { 이름: '연하진', 국어:92, 수학:98, 영어:96, 과학:98 } );
students.push( { 이름: '구지연', 국어:76, 수학:80, 영어:44, 과학:66 } );
students.push( { 이름: '구숙정', 국어:78, 수학:80, 영어:92, 과학:69 } );
for (var i in students) {
students[i].getSum = function( ) {
return this.국어 + this.수학 + this.영어 + this.과학;
};
students[i].getAverage = function( ) {
return this.getSum( ) / 4;
};
}
var output = '이름\\t총점\\t평균\\n';
for ( var i in students) {
with ( students[i] ) {
output+= 이름 + '\\t' + getSum( ) +'\\t' + getAverage( ) + '\\n';
}
}
alert(output);
var maxTotal = 0, name = '';
for (var i in students){
with(students[i]){
if (maxTotal<getSum()){ // students[i].getSum( )
name = 이름; // students[i].이름
maxTotal = getSum(); // students[i].getSum( )
}
}
}
alert(`1등은 ${name}입니다.`);
</script>
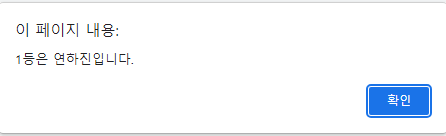
배열의 전개 연산자
얕은 복사
- 배열과 객체를 할당할 때 “얕은 복사”가 됨
<script>
const product0 = ['우유', '식빵']
const product1 = product0
product1.push('고구마')
product1.push('토마토')
product0.push('감자')
product0.push('사이다')
console.log('product0 :', product0)
console.log('product1 :', product1)
</script>
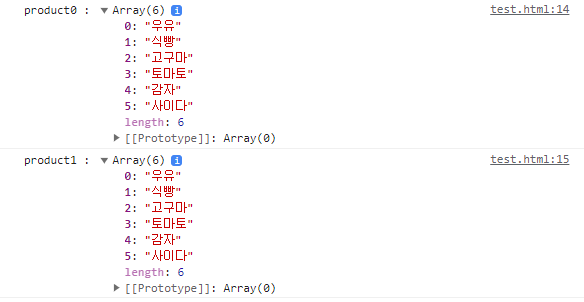
- 배열은 복사를 해도 다른 이름이 붙을뿐 그 값은 같다. 이러한 것을 “얕은 복사”(참조 복사)라고 함
- 얕은 복사와 달리 “깊은 복사”라는 복사가 있음(우리가 보통 알고 있는 복사)
깊은 복사
- 복사된 배열과 원본이 완전히 독립적인 복사
<script>
const product0 = ['우유', '식빵']
const product1 = [...product0]
product1.push('고구마')
product1.push('토마토')
console.log('product0 :', product0)
console.log('product1 :', product1)
</script>
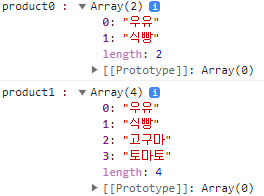
<script>
const product0 = ['우유', '식빵']
const product1 = ['고구마', ...product0, '토마토']
console.log('product0 :', product0)
console.log('product1 :', product1)
</script>
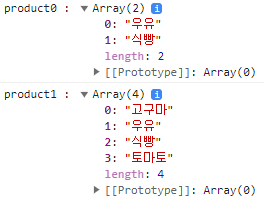
객체의 전개 연산자
얕은 복사
<script>
const dog = {이름:'구름',나이:4,종:'강아지'}
const newDog = dog
newDog.이름 = '별'
newDog.나이 = 5
console.log('dog :', dog)
console.log('newDog :', newDog)
</script>
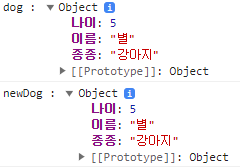
깊은 복사
<script>
const dog = {이름:'구름',나이:4,종:'강아지'}
const newDog = {...dog}
newDog.이름 = '별'
newDog.나이 = 5
console.log('dog :', dog)
console.log('newDog :', newDog)
</script>
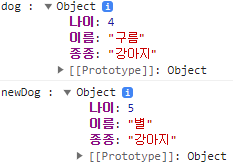
<script>
const dog = {이름:'구름',나이:4,종:'강아지'}
const newDog = {
...dog,
이름 = '별', //기존 속성 덮어쓰기
나이 = 5, //기존 속성 덮어쓰기
예방접종 : true
}
console.log('dog :', dog)
console.log('newDog :', newDog)
</script>
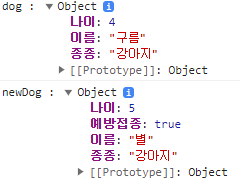
'빅데이터 분석가 양성과정 > JavaScript' 카테고리의 다른 글
문서 객체 모델 (0) | 2024.07.10 |
---|---|
브라우저 객체 모델 (0) | 2024.07.10 |
함수 (0) | 2024.07.10 |
반복문 (0) | 2024.07.10 |
조건문 (0) | 2024.07.10 |
객체
배열
- ‘인덱스’와 ‘요소’
- 접근 - 배열이름[인덱스]
- <script> var array =[‘사과’, ‘바나나’, ‘망고’, ‘딸기’]; alert( array[0] ) alert( array[1] ) </script>
객체
- 중괄호({})를 사용하여 생성
- 키와 요소
- 접근 - 객체이름[’키’] 또는 이름.키
- product[’유형’]
- product.유형
<script>var product = {제품명 : ‘7D 건조 망고‘,유형 : ‘당절임‘,성분: ‘망고, 설탕, 메타중아황산나트륨’,원산지: ‘필리핀‘};</script>
속성과 메서드
- 객체의 속성이 가질 수 있는 자료형
- 배열의 내부에 저장된 각각의 정보를 ‘요소’라고 부름
- 객체 내부에 저장된 각각의 정보를 ‘속성(property)’이라고 함
- 사물의 특성
- 객체의 속성 중 함수 자료형인 속성은 특별히 메서드(method)라 부름
- 사물의 동작
<script>var object = {number : 273,string : ‘ha ha’,boolean: ture,array : [52, 273, 103, 32],method : function ( ) { }};</script><script>var person = {name : ‘jaeyeong’,eat : function( food ) {alert(‘eat function’);}};person.eat( );</script>
- this 는 자기 자신, 파이썬에서 self와 같음
- 메서드 내에서 자기 자신(객체)이 가진 속성을 출력(속성에 접근)하고 싶을 때 자신이 가진 속성임을 분명하기 표시
- 자바스크립트에서는 this 키워드를 생략할 수 없음
<script> var person = { name : ‘jaeyeong’, eat : function( food ) { alert( this.name + ‘이가 ’ + food +’를(을) 먹습니다.’ ); } }; person.eat(‘밥’);</script>
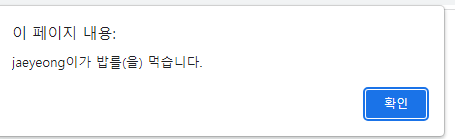
속성 존재 여부 확인
- 객체에 없는 속성에 접근하면 undefined 자료형이 나옴.
- 따라서 조건문으로 접근하려는 속성이 undefined인지 아닌지 확인하면 속성 존재 여부를 확인할 수 있음
<script> const obj = { name:'혼자 공부하는 파이썬', price:18000, publisher:'한빛미디어' } if(obj.name != undefined) { console.log('name 속성이 있음') } else { console.log('name 속성이 없음') }</script>

객체와 반복문
- 배열은 단순 for 문과 for in 문으로 요소에 쉽게 접근 가능.
- 객체는 단순 for 문으로 객체의 속성을 살펴 보는 것은 불가능하여
- for in 문을 사용 함
<script> var product = { name : ‘MicroSoft Visual Studio 2015 Ultimate’, price : ‘1,500,000원’, language : ‘한국어’, supportOS : ‘Win32/64’, subscription : true }; var output = ‘’; for( var key in product ) { output += key + ‘:’ + product[key] + ‘\\n’; } alert(output)</script>
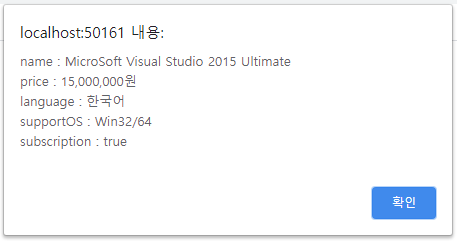
객체 관련 키워드
- 객체 관련 in 키워드와 with 키워드에 대해 살펴 봄
in 키워드
- 객체 내에 해당 속성이 있는지 확인
<script> var student = { 이름 : ‘이재영’, 국어: 92, 수학 : 98, 영어:96, 과학:98 }; var output =‘’; output += “ ’이름’ in student : “ + ( ‘이름’ in student ) + ‘\\n’; output += “ ’성별’ in student : “ + ( ‘성별’ in student ); alert(output);</script>
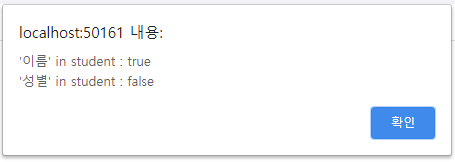
with 키워드
<script>var student = {이름 : ‘이재영’,국어점수: 92, 수학점수 : 98, 영어점수:96, 과학점수:98};var output =‘’with( student ) { output += ‘이름: ‘ + 이름 + ‘\\n’; output += ‘국어: ‘ + 국어점수 + ‘\\n’; output += ‘수학: ‘ + 수학점수 + ‘\\n’; output += ‘영어: ‘ + 영어점수 + ‘\\n’; output += ‘과학: ‘ + 과학점수+ ‘\\n’; output += ‘총점: ‘ + (국어점수 + 수학점수 + 영어점수 + 과학점수); }alert(output);</script>
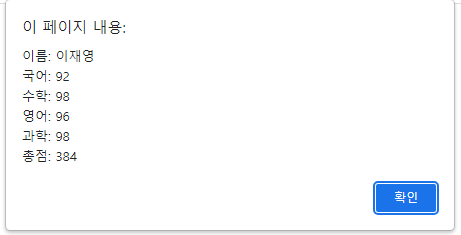
- 객체의 속성을 출력할 때 식별자(student)를 여러 번 사용하니 번거로움
- with( 객체 ) { <코드> }
- 객체를 지정하면 지정된 블럭 내에서 일일이 객체 명을 지정하지 않고 속성이름만 가지고 접근 가능
객체의 속성 추가와 제거
객체에 속성 추가
빈 객체 선언
<script> var student = { };</script>
동적으로 속성 추가
<script> var student = { }; student.이름 = ‘김성필‘; student.취미 = ‘낚시‘; student.특기 = ‘드라이브‘; student.장래희망 = ‘사장’;</script>
동적으로 메서드 추가
<script> var student = { }; student.이름 = ‘김성필‘; student.취미 = ‘낚시‘; student.특기 = ‘드라이브‘; student.장래희망 = ‘사장’; student.toString = function( ) { var output = ‘’; for( var key in this ) { if (key != ‘toString’) { output += key + ‘\\t’ + this[key] + ‘\\n’; } } return output; } alert( student.toString() );</script>
객체에 속성 제거
<script> var student = { }; student.이름 = ‘김성필‘; student.취미 = ‘낚시‘; student.특기 = ‘드라이브‘; student.장래희망 = ‘사장’; student.toString = function( ) { var output = ‘’; for( var key in this ) { if (key != ‘toString’) { output += key + ‘\\t’ + this[key] + ‘\\n’; } } return output; } alert( student.toString( ) ); delete( student.특기); alert( student.toString( ) ); </script>
메서드 간단 선언 구문
<script> const pet = { name : '구름', eat : function(food) { console.log(this.name +'은/는 ' + food +'을/를 먹습니다.') } } pet.eat('밥')</script>
<script> const pet = { name : '구름', eat(food) { console.log(this.name +'은/는 ' + food +'을/를 먹습니다.') } } pet.eat('밥')</script>
Number 객체
- 소수점 이하 몇 자리까지 남길 것인지 결정
<script> let n = 123.456789 n.toFixed(2) console.log(n) //⇒ n은 바뀌지 않음 n = n.toFixed(2) //=> 이렇게 해야 바뀜 console.log(n)</script>
NaN과 Infinity 확인하기 : isNaN( ), isFinity( )
Number.isNaN( ), Number.isFinity( )
<script> const n = Number('숫자로 변환 불가') console.log(n) console.log(Number.isNaN(n))</script>

<script> const n = 10 / 0 const m = -10 / 0 console.log(n) console.log(m) console.log(Number.isFinite(n)) console.log(Number.isFinite(m)) console.log( n === Infinity || n === -Infinity)</script>
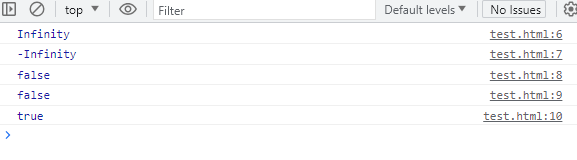
String 객체
문자열 양쪽 끝의 공백 없애기 : trim( )
<script> const stringA = '\\n 메시지를 입력하다 보니 ' console.log('kim') console.log('lee') console.log(stringA) console.log('trim() 적용') console.log(stringA.trim())</script>
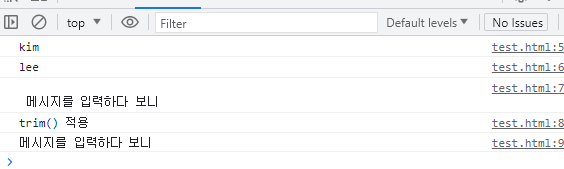
문자열을 특정 기호로 자르기: split( )
<script> const string = '수박, 사과, 복숭아, 참외' console.log(string.split(','))</script>
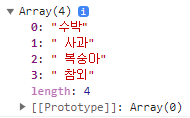
<script> const string = '수박, 사과, 복숭아, 참외' console.log(string.split(',').map(function(value){ return value.trim() }))</script>
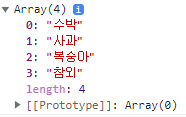
<script> const string = '수박, 사과, 복숭아, 참외' console.log(string.split(',').map((value)=>value.trim())) </script>
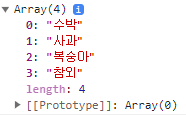
Quiz - 주어진 문자열의 각 행을 배열로 바꾸는 코드를 작성
let input = `일자, 달러, 엔, 유로02, 1141.8, 1097.46, 1262.3703, 1148.7, 1111.36, 1274.6504, 1140.6, 1107.81, 1266.58`
// 1<script> let input = ` 일자, 달러, 엔, 유로 02, 1141.8, 1097.46, 1262.37 03, 1148.7, 1111.36, 1274.65 04, 1140.6, 1107.81, 1266.58 ` let arr = input.split('\\n').map((value)=>value.trim()) console.log(arr); let filtered_lines = arr.filter(function(value){ return Boolean(value) }) console.log(filtered_lines) let output = filtered_lines.map(function(value){ return value.split(',') }) console.log(output) </script>
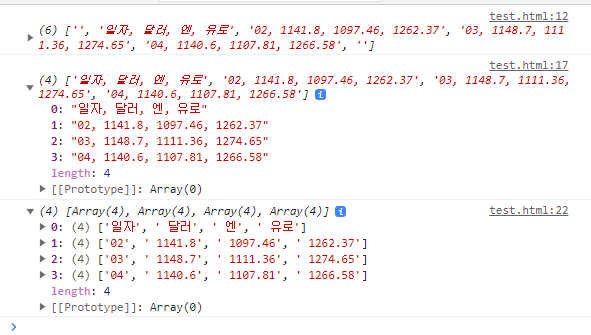
// 2<script> let input = ` 일자, 달러, 엔, 유로 02, 1141.8, 1097.46, 1262.37 03, 1148.7, 1111.36, 1274.65 04, 1140.6, 1107.81, 1266.58 ` let lines = input.trim() console.log(lines) lines = lines.split('\\n') console.log(lines) lines = lines.map(function(value){ return value.trim() }) console.log(lines) lines = lines.map(function(value){ return value.split(',') }) console.log('최종 결과물 : ', lines) // console.log('최종 결과물 : ', input.trim().split('\\n').map((value) => value.trim()).map((value)=>value.split(','))) </script>
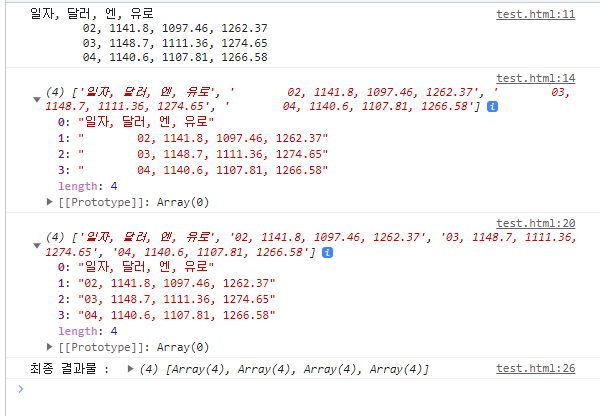
JSON 객체
- 인터넷에서 문자열로 데이터를 주고 받을 때는 CSV, XML, CSON 등의 다양한 자료 표현 방식이 있음
- 현재 가장 많이 사용되는 자료 표현 방식은 JSON 임
- JSON은 JavaScript Object Notation의 약자로 자바스크립트의 객체처럼 자료를 표현하는 방식
- JSON를 사용하여 ‘책’ 객체를 표현
- 하나의 자료를 표현 하는 예
{"name":"혼자 공부하는 자바스크립트","price":18000,"publisher":"한빛미디어"}
[{"name":"혼자 공부하는 자바스크립트","price":18000,"publisher":"한빛미디어"},{"name":"HTML5 웹 프로그래밍 입문","price":26000,"publisher":"한빛미디어"}]
- JSON은 “자바스크립트의 배열과 객체 표기 방식으로 표현된 데이터”임 - 추가적인 제약 조건이 있음
- 값을 표현할 때는 문자열, 숫자, 불 자료형만 사용(함수 등은 사용 불가)
- 문자열은 반드시 큰따옴표로 만듦
- 키(key)에도 반드시 따옴표를 붙여야 함
JSON.stringify( )
- 자바스크립트 객체를 JSON 문자열로 변환
<script> const data = [{ name :'혼자 공부하는 자바스크립트', price : 18000, publisher :'한빛미디어' },{ name : 'HTML5 웹 프로그래밍 입문', price : 26000, publisher :'한빛미디어' }] console.log(JSON.stringify(data)) console.log(JSON.stringify(data, null, 2))</script>
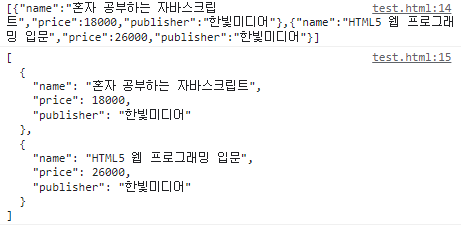
JSON.parse( )
- JSON 문자열을 자바스크립트 자료형으로 변환
<script> const data = [{ name :'혼자 공부하는 자바스크립트', price : 18000, publisher :'한빛미디어' },{ name : 'HTML5 웹 프로그래밍 입문', price : 26000, publisher :'한빛미디어' }] const json = JSON.stringify(data) console.log(json) console.log(JSON.parse(json))</script>
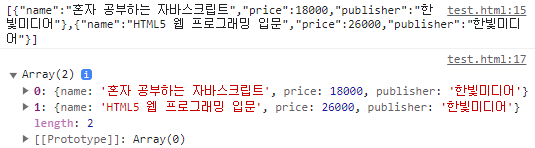
외부 스크립트 파일 읽어 들이기
<html> <head> <script src = "test.js"></script> <script> console.log('# main.html의 script 태그') console.log('sample의 값:', sample) </script> </head> <body> </body></html>
console.log('# test.js 파일')const sample = 10
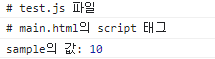
객체와 배열을 사용한 데이터 관리
학생들의 성적 총점과 평균을 계산하는 예제
- 객체의 필요한 속성을 추출하는 작업을 ‘추상화’라 함
<script> var student0 = { 이름: ‘윤인성’, 국어:87, 수학:98, 영어:88, 과학:95 }; var student1 = { 이름: ’연하진’, 국어:92, 수학:98, 영어:96, 과학:98 }; var student2 = { 이름: ‘구지연’, 국어:76, 수학:80, 영어:44, 과학:66 }; var student3 = { 이름: ‘구숙정’, 국어:78, 수학:80, 영어:92, 과학:69 };</script>
객체가 별로도 존재하면 관리가 어려움 → 각 객체를 배열의 요소로 할당
<script> var students = Array( ); students.push( { 이름: ‘윤인성’, 국어:87, 수학:98, 영어:88, 과학:95 } ); students.push( { 이름: ’연하진’, 국어:92, 수학:98, 영어:96, 과학:98 } ); students.push( { 이름: ‘구지연’, 국어:76, 수학:80, 영어:44, 과학:66 } ); students.push( { 이름: ‘구숙정’, 국어:78, 수학:80, 영어:92, 과학:69 } );</script>
- 배열 students에 4개의 요소가 생성됨
- 각각의 객체(여기서는 배열의 각 요소)에 getSum( )과 getAverage( ) 메서드를 부여
for (var i in students) { students[i].getSum = function( ) { return this.국어 + this.수학 + this.영어 + this.과학; }; students[i].getAverage = function( ) { return this.getSum( ) / 4; }; }
<script> var students = Array(); students.push( { 이름: '윤인성', 국어:87, 수학:98, 영어:88, 과학:95 } ); students.push( { 이름: '연하진', 국어:92, 수학:98, 영어:96, 과학:98 } ); students.push( { 이름: '구지연', 국어:76, 수학:80, 영어:44, 과학:66 } ); students.push( { 이름: '구숙정', 국어:78, 수학:80, 영어:92, 과학:69 } ); for (var i in students) { students[i].getSum = function( ) { return this.국어 + this.수학 + this.영어 + this.과학; }; students[i].getAverage = function( ) { return this.getSum( ) / 4; }; } var output = '이름\\t총점\\t평균\\n'; for ( var i in students) { with ( students[i] ) { output+= 이름 + '\\t' + getSum( ) +'\\t' + getAverage( ) + '\\n'; } } alert(output);</script>
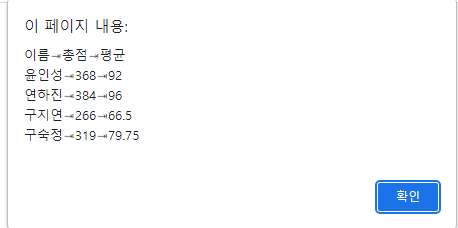
<script> var students = Array(); students.push( { 이름: '윤인성', 국어:87, 수학:98, 영어:88, 과학:95 } ); students.push( { 이름: '연하진', 국어:92, 수학:98, 영어:96, 과학:98 } ); students.push( { 이름: '구지연', 국어:76, 수학:80, 영어:44, 과학:66 } ); students.push( { 이름: '구숙정', 국어:78, 수학:80, 영어:92, 과학:69 } ); for (var i in students) { students[i].getSum = function( ) { return this.국어 + this.수학 + this.영어 + this.과학; }; students[i].getAverage = function( ) { return this.getSum( ) / 4; }; } var output = '이름\\t총점\\t평균\\n'; for ( var i in students) { with ( students[i] ) { output+= 이름 + '\\t' + getSum( ) +'\\t' + getAverage( ) + '\\n'; } } alert(output); var maxTotal = 0, name = ''; for (var i in students){ with(students[i]){ if (maxTotal<getSum()){ // students[i].getSum( ) name = 이름; // students[i].이름 maxTotal = getSum(); // students[i].getSum( ) } } } alert(`1등은 ${name}입니다.`); </script>
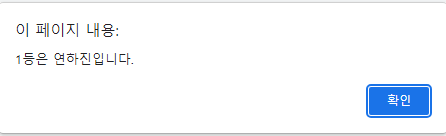
배열의 전개 연산자
얕은 복사
- 배열과 객체를 할당할 때 “얕은 복사”가 됨
<script> const product0 = ['우유', '식빵'] const product1 = product0 product1.push('고구마') product1.push('토마토') product0.push('감자') product0.push('사이다') console.log('product0 :', product0) console.log('product1 :', product1) </script>
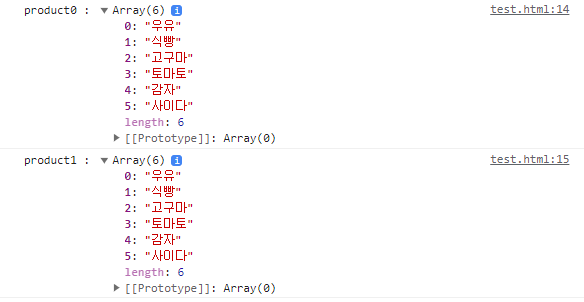
- 배열은 복사를 해도 다른 이름이 붙을뿐 그 값은 같다. 이러한 것을 “얕은 복사”(참조 복사)라고 함
- 얕은 복사와 달리 “깊은 복사”라는 복사가 있음(우리가 보통 알고 있는 복사)
깊은 복사
- 복사된 배열과 원본이 완전히 독립적인 복사
<script> const product0 = ['우유', '식빵'] const product1 = [...product0] product1.push('고구마') product1.push('토마토') console.log('product0 :', product0) console.log('product1 :', product1) </script>
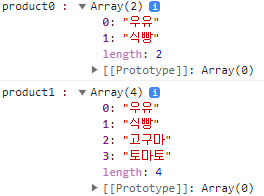
<script> const product0 = ['우유', '식빵'] const product1 = ['고구마', ...product0, '토마토'] console.log('product0 :', product0) console.log('product1 :', product1) </script>
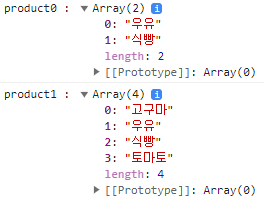
객체의 전개 연산자
얕은 복사
<script> const dog = {이름:'구름',나이:4,종:'강아지'} const newDog = dog newDog.이름 = '별' newDog.나이 = 5 console.log('dog :', dog) console.log('newDog :', newDog) </script>
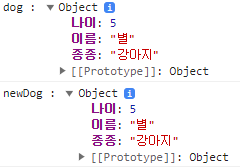
깊은 복사
<script> const dog = {이름:'구름',나이:4,종:'강아지'} const newDog = {...dog} newDog.이름 = '별' newDog.나이 = 5 console.log('dog :', dog) console.log('newDog :', newDog) </script>
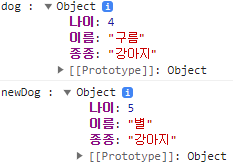
<script> const dog = {이름:'구름',나이:4,종:'강아지'} const newDog = { ...dog, 이름 = '별', //기존 속성 덮어쓰기 나이 = 5, //기존 속성 덮어쓰기 예방접종 : true } console.log('dog :', dog) console.log('newDog :', newDog) </script>
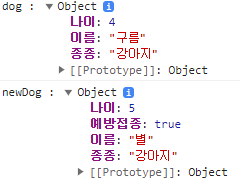
'빅데이터 분석가 양성과정 > JavaScript' 카테고리의 다른 글
문서 객체 모델 (0) | 2024.07.10 |
---|---|
브라우저 객체 모델 (0) | 2024.07.10 |
함수 (0) | 2024.07.10 |
반복문 (0) | 2024.07.10 |
조건문 (0) | 2024.07.10 |